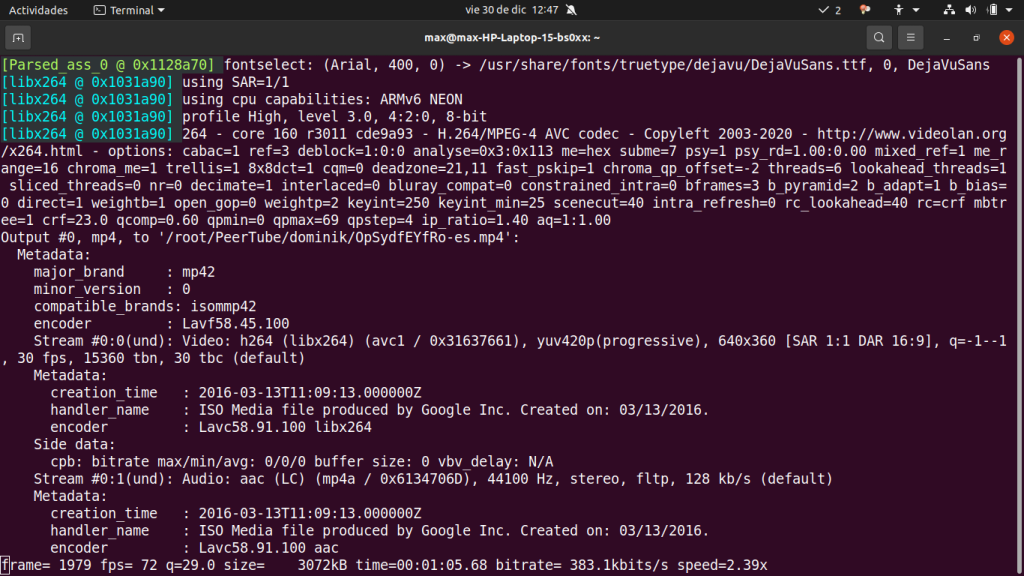
Aquí el script para bajar los videos desde youtube e insertarlos automáticamente en Peertube.
Esta versión corrige muchos fallos y se añade una característica muy importante:
Antes de bajar el vídeo, hace una comprobación para ver si ya hay uno con el mismo titulo en Peertube.
Creo que de momento es suficiente. Claro uue puede pasar que dos vídeos diferentes puedan tener el mismo título, pero esto no me ha ocurrido nunca. De todas formas más adelante voy añadir un parámetro mas para reducir esta posibilidad al mínimo.
Esta es la última versión que funcionará solo en la consola, la próxima espero que tenga una interfaz gráfica
#!/bin/bash
# Substitute wrapper for peertube-import-videos.js.
# This script uses youtube-dl and jq to download the youtube videos and upload them to your PeerTube instance
# using the peertube-upload.js script from the PeerTube CLI suite.
#
# Works on Debian10 Not tested on other distributions.
#
# sudo curl -L https://yt-dl.org/downloads/latest/youtube-dl -o /usr/local/bin/youtube-dl
# sudo chmod a+rx /usr/local/bin/youtube-dl
# sudo apt install jq -y
#
# I suggest you use "screen" to put the script in the background.
# Channel import can take many hours (or days) and if your session is via ssh, it could crash due network problems.
#
# Change variable fields
# IN="Channel URL or PlayList URL"
# resolution="18" # 18 = 360p (640x360 mp4) | 22 = 720p (1280x720 mp4)
# channel="PeerTube Channel" Please create it before and paste here the sanitize nambre without spaces and lowercase
# server="https://yourPeerTubeInstanceUrl"
# username="username of PeerTube Instance"
# password="yourPassword"
#
# embedded translated subtitles!!
# If you have a YouTube channel in Japanese and you want the videos with subtitles in Spanish, put "es" in "language"
#
# Possibility of using your browser's cookies.
# Cookies must be exported using the Firefox extension "cookies.txt".
# Once you have exported the yotube cookies from your browser, copy the cookies.txt file to the server where
# peertube works with the channel downloader. I put them in /root/cookies.txt, but you can use any other place.
#
# Possibility to use a proxy (to change your IP)
#
# please before run this script install the CLI tools following the documentation
# https://docs.joinpeertube.org/maintain-tools
# ... and then register a remote user with
# peertube auth add -u 'PEERTUBE_URL' -U 'PEERTUBE_USER' --password 'PEERTUBE_PASSWORD'
# peertube auth list.
# now the program will do a search in the Peertube database to find out if the video already exists.
# And if it exists, it doesn't download it.
#
# on 2022-12-30
# maxlinux2000@gmail.com
# max2k@preparandonos.es
#############################################################
channel="almacen3" # insert the Peertube channel name, like "profesorpardalbrasil" or "ea3grn"
IN="https://www.youtube.com/@26CW128Jake/videos" # Youtube url channel
resolution="18" # youtube-dl -F https://youtube<channel> to have the listo of the other avalaible resolutions
server="http://videos3.preparandonos.es" # change it!
username="max2k" # change it!
password="MyPassword" # change it!
## force embed subtitles in language of your choice, i.e.: fr es de it ru ch
## if empty no subtitles will be embedded
language="es"
# proxy
# proxy=' --proxy http://123.123.123.123:8080' #
# cookies form firefox extension "cookies.txt"
#cookies=" --cookies /root/cookies.txt"
PWD=$(pwd)
mkdir -p $channel
touch $PWD/$channel/$channel-yt-done
touch $channel/$channel-yt-list
###############################################################################################################################
###############################################################################################################################
# From here do not touch anything unless you know what you are doing.
RAMDISK="/dev/shm"
PeerHome=/root/PeerTube
PWD=$(pwd)
mkdir -p $PeerHome/$channel
touch $PeerHome/$channel/$channel-yt
# install jq
if [ -f /usr/bin/jq ]; then
echo "jq installed"
else
clear
echo "please install jq!
apt install jq
"
exit
fi
if [ -f /usr/local/bin/youtube-dl ]; then
echo "youtube-dl installed"
else
clear
echo "please install youtube-dl!
sudo curl -L https://yt-dl.org/downloads/latest/youtube-dl -o /usr/local/bin/youtube-dl
sudo chmod a+rx /usr/local/bin/youtube-dl
"
exit
fi
#####################################################################################################
#Funtions Zone start
processLog() {
id=$1
yt_code=$(echo $id | sed 's|^MINUS|-|g')
cat -- $PeerHome/$channel/$channel-yt-list | grep -v -- "$yt_code" > $PeerHome/$channel/tmp1
mv $PeerHome/$channel/tmp1 $PeerHome/$channel/$channel-yt-list
}
countdown() {
secs=60
while [ $secs -gt 0 ]; do
echo -ne "$secs\033[0K\r"
sleep 1
: $((secs--))
done
}
countdown600() {
secs=600
while [ $secs -gt 0 ]; do
echo -ne "$secs\033[0K\r"
sleep 1
: $((secs--))
done
}
query() {
:> $RAMDISK/videoExist
cd /
title="$1"
export PGPASSWORD=$(cat /var/www/peertube/config/production.yaml | grep -A6 "database:" | grep "password" | cut -d "'" -f2)
exist=$(psql -h 'localhost' -U "peertube" -d peertube_peertube -t -c "SELECT name FROM video where name = '$title'")
exist=$(echo $exist | sed 's|^ ||')
echo "----------------------------------------------------------------------"
echo "New Video title: $title"
echo "Found in database: $exist"
echo "----------------------------------------------------------------------"
if [ ! -z "$exist" ]; then
echo "true" > $RAMDISK/videoExist
else
echo "false" > $RAMDISK/videoExist
fi
cd $PeerHome/$channel/
}
standard() {
echo "#########################################################################################################"
id=$1
yt_code=$(echo $id | sed 's|^MINUS|-|g')
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing..."
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
############### End Detector ###########
############### Start downloader #######
youtube-dl $cookies $proxy --write-info-json --skip-download -o $PeerHome/$channel/$id "https://www.youtube.com/watch?v=$yt_code"
json=$(cat -- $PeerHome/$channel/$id.info.json)
title=$(echo $json | jq .fulltitle | tr -d '"')
query "$title"
VideoExist=$(cat $RAMDISK/videoExist)
if [ "$VideoExist" = "true" ]; then
echo "Title already donwloaded, skipping"
rm $RAMDISK/videoExist
processLog $id
else
while :
do
echo "Video not present, downloadind..."
youtube-dl $cookies $proxy --write-thumbnail -f "$resolution" -o $PeerHome/$channel/$id.mp4 "https://www.youtube.com/watch?v=$yt_code"
if [ ! -f $PeerHome/$channel/$id.mp4 ]; then
echo "internet is down or network problems... waiting 10 minuts"
countdown600
else
echo "following"
break
fi
done
json=$(cat -- $PeerHome/$channel/$id.info.json)
title=$(echo $json | jq .fulltitle | tr -d '"')
datejson=$(cat -- $PeerHome/$channel/$id.info.json | less | jq .upload_date | tr -d '"')
date=$(date -d $datejson +%Y-%m-%d)
file=$(ls $PeerHome/$channel/$id.mp4 2>/dev/null)
if [ -f $PeerHome/$channel/$id.jpg ]; then
thumb=$(ls $PeerHome/$channel/$id.jpg)
fi
if [ -f $PeerHome/$channel/$id.webp ]; then
thumb=$(ls $PeerHome/$channel/$id.webp)
fi
description=$(echo $json | jq .description | tr -d '"' | sed 's|\\n|\n|g')
lang=$(echo $json | jq '.language')
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing..."
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
cd $PeerHome
node $PeerHome/dist/server/tools/peertube-upload.js -n "$title" -C "$channel" -b $thumb -d "$date - $yt_code - $description" -L "$lang" -U "$username" -p "$password" -f "$file" -u $server
rm $file 2>/dev/null
rm $PeerHome/$channel/$id.* 2>/dev/null
fi
echo "@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@2"
}
subtitle() {
echo "#########################################################################################################"
id=$1
yt_code=$(echo $id | sed 's|^MINUS|-|g')
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing..."
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
############### End Detector ###########
############### Start downloader #######
youtube-dl $cookies $proxy --write-info-json --skip-download -o $PeerHome/$channel/$id "https://www.youtube.com/watch?v=$yt_code"
json=$(cat -- $PeerHome/$channel/$id.info.json)
title=$(echo $json | jq .fulltitle | tr -d '"')
query "$title"
VideoExist=$(cat $RAMDISK/videoExist)
if [ "$VideoExist" = "true" ]; then
echo "Title already donwloaded, skipping"
rm $RAMDISK/videoExist
processLog $id
else
#### Start FFMPEG embeder Subtitles and Uploader ####
youtube-dl $cookies $proxy --write-info-json --write-thumbnail -f "$resolution" -o $PeerHome/$channel/$id.mp4 "https://www.youtube.com/watch?v=$yt_code"
# youtube-dl $cookies $proxy --write-info-json --write-thumbnail -f "$resolution" --write-auto-sub --sub-lang $language -o "$PWD/$channel/$id.mp4" "https://www.youtube.com/watch?v=$yt_code" -o $PWD/$channel/$id.$language.vtt
youtube-dl $cookies $proxy --write-info-json --write-thumbnail -f "$resolution" --write-auto-sub --sub-lang $language --skip-download "https://www.youtube.com/watch?v=$yt_code" -o $PeerHome/$channel/$id.$language.vtt
if [ ! -f $PeerHome/$channel/$id.$language.vtt ]; then
youtube-dl $cookies --write-sub --sub-lang $language --skip-download "https://www.youtube.com/watch?v=$yt_code" -o $PeerHome/$channel/$id.$language.vtt
fi
file=$PeerHome/$channel/$id-$language.mp4
json=$(cat -- $PeerHome/$channel/$id.$language.vtt.info.json)
title=$(echo $json | jq .fulltitle | tr -d '"')
datejson=$(cat -- $PeerHome/$channel/$id.$language.vtt.info.json | less | jq .upload_date | tr -d '"')
date=$(date -d $datejson +%Y-%m-%d)
# echo file=$file
description=$(echo $json | jq .description | tr -d '"' | sed 's|\\n|\n|g')
if [ -f $id.jpg ]; then
thumb=$(ls $PeerHome/$channel/$id.jpg)
fi
if [ -f $PeerHome/$channel/$id.webp ]; then
thumb=$(ls $PeerHome/$channel/$id.webp)
fi
# lang=$(echo $json | jq . | grep "lang=" | head -n1 | cut -d '?' -f2 | cut -d '&' -f1 | sed 's|lang=||g')
lang=$(echo $json | jq '.language')
if [ -f $PeerHome/$channel/$id.$language.vtt.$language.vtt ]; then
echo "-----------------------
Lang=$lang
"
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing..."
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
############### End Detector ###########
ffmpeg -hide_banner -threads 1 -i "$PeerHome/$channel/$id.$language.vtt.$language.vtt" $PeerHome/$channel/sub.ass -y
ffmpeg -hide_banner -threads 1 -i "$PeerHome/$channel/$id.mp4" -vf "ass=$PeerHome/$channel/sub.ass" -empty_hdlr_name 1 "$PeerHome/$channel/$id-$language.mp4" -y
rm $PeerHome/$channel/sub.ass $PeerHome/$channel/$id.$language.vtt.$language.vtt $PeerHome/$channel/$id.mp4
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing...1"
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
############### End Detector ###########
if [ -f $PeerHome/$channel/$id.webp ]; then
thumb=$(ls $PeerHome/$channel/$id.webp)
fi
cd $PeerHome
node $PeerHome/dist/server/tools/peertube-upload.js -n "$title" -C "$channel" -b $thumb -d "$date - $yt_code - $description" -L "$lang" -U "$username" -p "$password" -f "$file" -u $server
rm $PeerHome/$channel/$id.mp4 $PeerHome/$channel/$id-$language.mp4 $PeerHome/$channel/$id.info.json $PeerHome/$channel/$id.jpg $PeerHome/$channel/$id.webp 2>/dev/null
echo "----------------------"
else
echo "-----------------------
Lang=en
skipping. Please at the end, open the $channel-yt-2-translate.txt file go to youtube and generate the translation
whatching the videos (all) with localized subtitles. Then repeat the process.
"
file=$PeerHome/$channel/$id.mp4
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing...2"
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
############### End Detector ###########
cd $PeerHome
node $PeerHome/dist/server/tools/peertube-upload.js -n "$title" -C "$channel" -b $thumb -d "$date - $yt_code - $description" -L "$lang" -U "$username" -p "$password" -f "$file" -u "$server"
rm $PeerHome/$channel/$id.mp4 $PeerHome/$channel/$id-$language.mp4 $PeerHome/$channel/$id.info.json $PeerHome/$channel/$id.jpg $PeerHome/$channel/$id.webp 2>/dev/null
echo $yt_code >> $PeerHome/$channel-yt-2-translate.txt
cat $PeerHome/$channel-yt-2-translate.txt | sort | uniq > tmp2
mv tmp2 $PeerHome/$channel-yt-2-translate.txt
echo "----------------------"
fi
# OLD ###################################################################################
# while :
# do
# echo "Video not present, downloadind..."
# youtube-dl $cookies $proxy --write-thumbnail -f "$resolution" -o $PeerHome/$channel/$id.mp4 "https://www.youtube.com/watch?v=$yt_code"
# if [ ! -f $PeerHome/$channel/$id.mp4 ]; then
# echo "internet is down or network problems... waiting 10 minuts"
# countdown600
# else
# echo "following"
# break
# fi
# done
# json=$(cat -- $PeerHome/$channel/$id.info.json)
# title=$(echo $json | jq .fulltitle | tr -d '"')
# datejson=$(cat -- $PeerHome/$channel/$id.info.json | less | jq .upload_date | tr -d '"')
# date=$(date -d $datejson +%Y-%m-%d)
# file=$(ls $PeerHome/$channel/$id.mp4 2>/dev/null)
# if [ -f $PeerHome/$channel/$id.jpg ]; then
# thumb=$(ls $PeerHome/$channel/$id.jpg)
# fi
# if [ -f $PeerHome/$channel/$id.webp ]; then
# thumb=$(ls $PeerHome/$channel/$id.webp)
# fi
# description=$(echo $json | jq .description | tr -d '"' | sed 's|\\n|\n|g')
# lang=$(echo $json | jq '.language')
# ##### ffmpeg activity detector #######
# while :
# do
# FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
# if [ -z $FF ]; then
# echo "FFMPEG is not active, continuing..."
# break
# else
# echo "FFMPEG is running: waiting 60 secs"
# countdown
# fi
# done
# cd $PeerHome
# node $PeerHome/dist/server/tools/peertube-upload.js -n "$title" -C "$channel" -b $thumb -d "$date - $yt_code - $description" -L "$lang" -U "$username" -p "$password" -f "$file" -u $server
# rm $file 2>/dev/null
# rm $PeerHome/$channel/$id.* 2>/dev/null
fi
echo "@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@@2"
}
subtitleStop() {
id=$1
echo id=$id
yt_code=$(echo $id | sed 's|^MINUS|-|g')
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing..."
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
#### start downloader #####
while :
do
echo youtube-dl $cookies $proxy --write-info-json --write-thumbnail -f "$resolution" -o $PeerHome/$channel/$id.mp4 "https://www.youtube.com/watch?v=$yt_code"
echo youtube-dl $cookies $proxy --write-info-json --write-thumbnail -f "$resolution" --write-auto-sub --sub-lang $language --skip-download "https://www.youtube.com/watch?v=$yt_code" -o $PeerHome/$channel/$id.$language.vtt
youtube-dl $cookies $proxy --write-info-json --write-thumbnail -f "$resolution" -o $PeerHome/$channel/$id.mp4 "https://www.youtube.com/watch?v=$yt_code"
youtube-dl $cookies $proxy --write-info-json --write-thumbnail -f "$resolution" --write-auto-sub --sub-lang $language --skip-download "https://www.youtube.com/watch?v=$yt_code" -o $PeerHome/$channel/$id.$language.vtt
if [ ! -f $PeerHome/$channel/$id.$language.vtt ]; then
youtube-dl $cookies --write-sub --sub-lang $language --skip-download "https://www.youtube.com/watch?v=$yt_code" -o $PeerHome/$channel/$id.$language.vtt
fi
if [ ! -f $PeerHome/$channel/$id.mp4 ]; then
echo "internet is down or network problems... waiting 10 minuts"
countdown600
else
echo "following"
break
fi
done
#### end downloader ######
#### Start FFMPEG embeder Subtitles and Uploader ####
file=$PeerHome/$channel/$id-$language.mp4
json=$(cat -- $PeerHome/$channel/$id.$language.vtt.info.json)
title=$(echo $json | jq .fulltitle | tr -d '"')
datejson=$(cat -- $PeerHome/$channel/$id.$language.vtt.info.json | less | jq .upload_date | tr -d '"')
date=$(date -d $datejson +%Y-%m-%d)
# echo file=$file
description=$(echo $json | jq .description | tr -d '"' | sed 's|\\n|\n|g')
if [ -f $id.jpg ]; then
thumb=$(ls $PeerHome/$channel/$id.jpg)
fi
if [ -f $PeerHome/$channel/$id.webp ]; then
thumb=$(ls $PeerHome/$channel/$id.webp)
fi
# lang=$(echo $json | jq . | grep "lang=" | head -n1 | cut -d '?' -f2 | cut -d '&' -f1 | sed 's|lang=||g')
lang=$(echo $json | jq '.language')
if [ -f $PeerHome/$channel/$id.$language.vtt.$language.vtt ]; then
echo "-----------------------
Lang=$lang
"
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing..."
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
############### End Detector ###########
ffmpeg -hide_banner -threads 1 -i "$PeerHome/$channel/$id.$language.vtt.$language.vtt" $PeerHome/$channel/sub.ass -y
ffmpeg -hide_banner -threads 1 -i "$PeerHome/$channel/$id.mp4" -vf "ass=$PeerHome/$channel/sub.ass" -empty_hdlr_name 1 "$PeerHome/$channel/$id-$language.mp4" -y
rm $PeerHome/$channel/sub.ass $PeerHome/$channel/$id.$language.vtt.$language.vtt $PeerHome/$channel/$id.mp4
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing..."
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
############### End Detector ###########
node $PeerHome/dist/server/tools/peertube-upload.js -n "$title" -C "$channel" -b $thumb -d "$date - $yt_code - $description" -L "$lang" -U "$username" -p "$password" -f "$file" -u $server
rm $PeerHome/$channel/$id.mp4 $PeerHome/$channel/$id-$language.mp4 $PeerHome/$channel/$id.info.json $PeerHome/$channel/$id.jpg $PeerHome/$channel/$id.webp 2>/dev/null
echo "----------------------"
else
echo "-----------------------
Lang=en
skipping. Please at the end, open the $channel-yt-2-translate.txt file go to youtube and generate the translation
whatching the videos (all) with localized subtitles. Then repeat the process.
"
file=$PeerHome/$channel/$id.mp4
##### ffmpeg activity detector #######
while :
do
FF=$(ps -A | grep "ffmpeg" | tail -n 1 | tr -d ' ' | tr -d ':')
if [ -z $FF ]; then
echo "FFMPEG is not active, continuing..."
break
else
echo "FFMPEG is running: waiting 60 secs"
countdown
fi
done
############### End Detector ###########
node $PeerHome/dist/server/tools/peertube-upload.js -n "$title" -C "$channel" -b $thumb -d "$date - $yt_code - $description" -L "$lang" -U "$username" -p "$password" -f "$file" -u "$server"
rm $PeerHome/$channel/$id.mp4 $PeerHome/$channel/$id-$language.mp4 $PeerHome/$channel/$id.info.json $PeerHome/$channel/$id.jpg $PeerHome/$channel/$id.webp 2>/dev/null
echo $yt_code >> $channel-yt-2-translate.txt
cat $channel-yt-2-translate.txt | sort | uniq > tmp2
mv tmp2 $channel-yt-2-translate.txt
echo "----------------------"
fi
}
#touch ./$channel-yt-done
#:> $channel-yt-list
### old #youtube-dl --cookies $cookies -u $yt_user -p $yt_pass --verbose $proxy --playlist-reverse --get-id $IN | tee -a $channel-yt-list
#youtube-dl --cookies $cookies --verbose $proxy --playlist-reverse --get-id $IN | tee -a $channel-yt-list
#cat $channel-yt-list | uniq > tmp
#mv tmp $channel-yt-list
#######################################################################################################
# Start Program
#cd $channel
#ls *.part | cut -d '.' -f1 > tmp
#for i in `cat tmp`; do
# sed -i "s/$i//g" $channel-yt-done
# sed -i "s/$i//g" $channel-yt-list
# sed -i "1s/^/$i\n/" $channel-yt-list
#done
#rm tmp
#cd ..
if [ ! -s $PeerHome/$channel/$channel-yt-list ]; then
echo "File $channel-yt-list IS EMPTY"
#### start LIST downloader #####
youtube-dl $cookies --verbose $proxy --playlist-reverse --get-id $IN | tee -a $PeerHome/$channel/$channel-yt-list
cp $PeerHome/$channel/$channel-yt-list $PeerHome/$channel/$channel-yt-list.orig
#### end LIST downloader ######
cat $PeerHome/$channel/$channel-yt-list | uniq > $PeerHome/$channel/tmp
mv $PeerHome/$channel/tmp $PeerHome/$channel/$channel-yt-list
else
echo "File $channel-yt-list HAS SOMETHING"
fi
for id in $(cat -- $PeerHome/$channel/$channel-yt-list); do
echo processing $id
id=$(echo $id | sed 's|^-|MINUS|g')
if [ -z $language ]; then
standard $id
else
subtitle $id
fi
echo "-------------------------"
done
cd $PeerHome/$channel/
rm *.mp4 *.webp *.jpg *.info.json *.vtt 2>/dev/null